Integrating MongoDB with Next.js
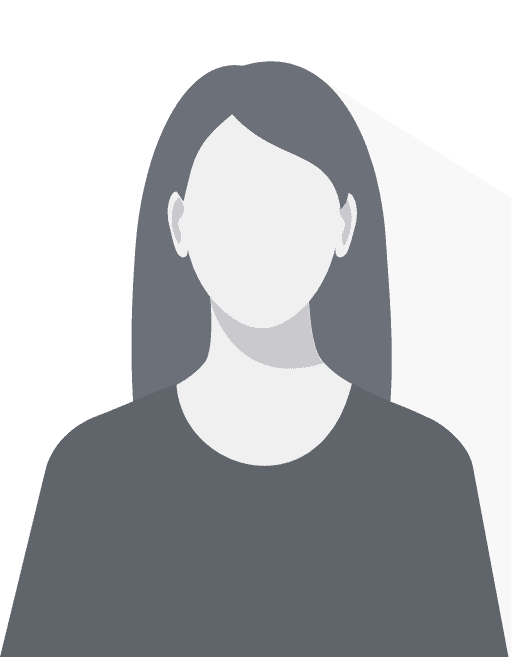
Muskan
March 21, 2025
Integrating MongoDB with Next.js
MongoDB is a popular NoSQL database that works great with Next.js applications. In this guide, we'll explore how to integrate MongoDB with your Next.js project.
Setting Up MongoDB
First, you'll need to set up a MongoDB database. You can use MongoDB Atlas, which provides a free tier for small projects.
- Create an account on MongoDB Atlas
- Create a new cluster
- Set up database access (username and password)
- Set up network access (IP whitelist)
- Get your connection string
Installing Dependencies
Install the required packages in your Next.js project:
npm install mongodb mongoose
Creating a Connection Utility
Create a utility file to handle the MongoDB connection:
// lib/mongodb.js
import mongoose from 'mongoose';
const MONGODB_URI = process.env.MONGODB_URI;
if (!MONGODB_URI) {
throw new Error('Please define the MONGODB_URI environment variable');
}
let cached = global.mongoose;
if (!cached) {
cached = global.mongoose = { conn: null, promise: null };
}
async function connectToDatabase() {
if (cached.conn) {
return cached.conn;
}
if (!cached.promise) {
cached.promise = mongoose.connect(MONGODB_URI).then((mongoose) => {
return mongoose;
});
}
cached.conn = await cached.promise;
return cached.conn;
}
export default connectToDatabase;
Creating a Model
Define a Mongoose model for your data:
// models/Post.js
import mongoose from 'mongoose';
const PostSchema = new mongoose.Schema({
title: {
type: String,
required: true,
},
content: {
type: String,
required: true,
},
createdAt: {
type: Date,
default: Date.now,
},
});
export default mongoose.models.Post || mongoose.model('Post', PostSchema);
Creating API Routes
Create API routes to interact with your MongoDB database:
// pages/api/posts/index.js
import connectToDatabase from '../../../lib/mongodb';
import Post from '../../../models/Post';
export default async function handler(req, res) {
await connectToDatabase();
if (req.method === 'GET') {
// Get all posts
const posts = await Post.find({}).sort({ createdAt: -1 });
res.status(200).json({ success: true, data: posts });
} else if (req.method === 'POST') {
// Create a new post
const post = await Post.create(req.body);
res.status(201).json({ success: true, data: post });
} else {
res.status(405).json({ success: false, message: 'Method not allowed' });
}
}
Using Data in Your Pages
Fetch and display data from MongoDB in your Next.js pages:
// pages/index.js
import { useEffect, useState } from 'react';
export default function Home() {
const [posts, setPosts] = useState([]);
useEffect(() => {
async function fetchPosts() {
const res = await fetch('/api/posts');
const { data } = await res.json();
setPosts(data);
}
fetchPosts();
}, []);
return (
<div>
<h1>Blog Posts</h1>
{posts.map((post) => (
<div key={post._id}>
<h2>{post.title}</h2>
<p>{post.content}</p>
</div>
))}
</div>
);
}
Conclusion
Integrating MongoDB with Next.js provides a powerful solution for building full-stack applications. With server-side rendering and API routes, Next.js makes it easy to create applications that interact with your MongoDB database.