CSS Grid Layout: A Complete Guide
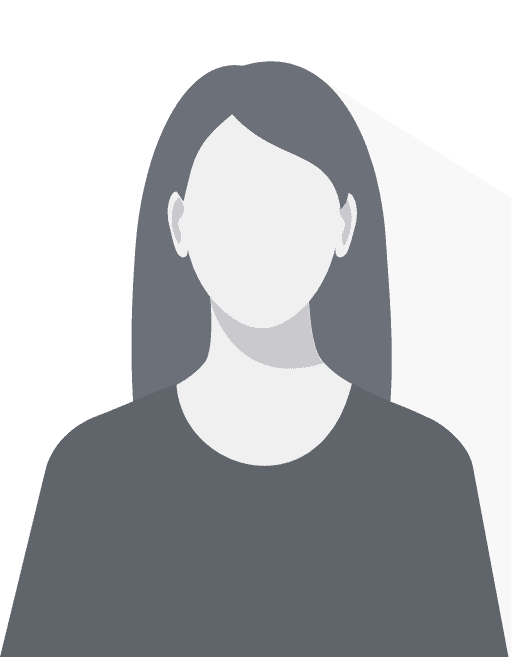
Muskan
March 21, 2025
CSS Grid Layout: A Complete Guide
CSS Grid Layout is a powerful two-dimensional layout system designed for the web. It allows you to create complex grid-based layouts with ease.
Basic Concepts
CSS Grid Layout introduces several new concepts:
- Grid Container: The element on which
display: grid
is applied. - Grid Items: The direct children of the grid container.
- Grid Lines: The horizontal and vertical lines that divide the grid.
- Grid Tracks: The space between two adjacent grid lines (rows or columns).
- Grid Cell: The intersection of a row and a column.
- Grid Area: A rectangular area bounded by four grid lines.
Creating a Grid
To create a grid container, you simply need to set the display
property to grid
:
.container {
display: grid;
grid-template-columns: 1fr 1fr 1fr;
grid-template-rows: 100px 200px;
gap: 20px;
}
This creates a 3-column, 2-row grid with a 20px gap between grid items.
Placing Items
You can place items in the grid using various properties:
.item {
grid-column: 1 / 3; /* Start at line 1, end at line 3 */
grid-row: 1 / 2; /* Start at line 1, end at line 2 */
}
Or using the shorthand:
.item {
grid-area: 1 / 1 / 2 / 3; /* row-start / column-start / row-end / column-end */
}
Responsive Grids
CSS Grid makes it easy to create responsive layouts:
.container {
display: grid;
grid-template-columns: repeat(auto-fill, minmax(250px, 1fr));
gap: 20px;
}
This creates a grid with as many columns as can fit, where each column is at least 250px wide.
Grid Template Areas
You can name areas of your grid and place items in those areas:
.container {
display: grid;
grid-template-columns: 1fr 3fr 1fr;
grid-template-rows: auto 1fr auto;
grid-template-areas:
"header header header"
"sidebar content aside"
"footer footer footer";
}
.header { grid-area: header; }
.sidebar { grid-area: sidebar; }
.content { grid-area: content; }
.aside { grid-area: aside; }
.footer { grid-area: footer; }
Browser Support
CSS Grid is supported in all modern browsers. For older browsers, you can use feature detection and provide fallbacks:
@supports (display: grid) {
/* Grid styles */
}
@supports not (display: grid) {
/* Fallback styles */
}
Conclusion
CSS Grid Layout is a powerful tool for creating complex web layouts. It provides a level of control that was previously difficult to achieve with CSS. By understanding its concepts and properties, you can create sophisticated, responsive designs with less code and greater flexibility.